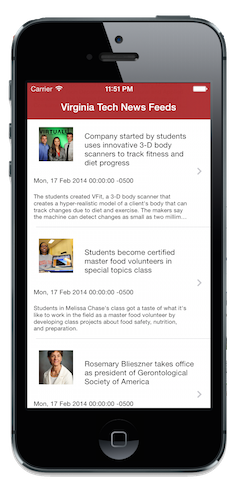
I have used grand central dispatch before, but never NSURLConnections. I have had problems with loading images on to a simple table view for one of my old tutorial projects. Today I decided to dust VTFeeds (application) off the shelf and try to fix the problem. With iOS 7 coming out with a new network's framework. Apple has replaced NSURLConnections with NSURLSessions. This was the perfect opportunity to explore how they work. According to Apple's documentation:
The NSURLSession class and related classes provide an API for downloading content via HTTP. This API provides a rich set of delegate methods for supporting authentication and gives your app the ability to perform background downloads when your app is not running or, in iOS, while your app is suspended.
So with NSURLSessions it's actually perfect, we don't really need to worry about creating our own background threads to perform background downloading. Now NSURLSession does all this for you!
It's actually really simple to do. We first create an NSURLConfiguration. For the time being since we don't need to really specify any configs we will leave it as default. Secondly we create a NSURLSession and set up the delegate for this class. Third we use the session we created to create a task. In this case we create an NSURLSessionDownloadTask. When the download task is complete, it saves the data into a temporary file path, in which you must retrieve.
Since all user interface operations are done on the main thread, we set the image we downloaded in a UIImageView within the main thread. I also currently place the downloaded images in an NSMutableDictionary which acts as a cache for the images. Maybe i'll explore NSURLCache some other time. :)
Solution
NSURL *URL = [NSURL URLWithString:currentArticle.imageURL];
//First create an NSURLConfiguration
[NSURLSessionConfiguration defaultSessionConfiguration];
//Creates a session thatt conforms to the current class as a delegate.
NSURLSession *session =
[NSURLSession sessionWithConfiguration:sessionConfiguration
delegate:self
delegateQueue:nil];
//Session creates the Download Task.
NSURLSessionDownloadTask *getImageTask =
[session downloadTaskWithURL:URL
completionHandler:^(NSURL *location,
NSURLResponse *response,
NSError *error) {
UIImage *imageDownloaded =
[UIImage imageWithData:
[NSData dataWithContentsOfURL:location]];
dispatch_async(dispatch_get_main_queue(), ^{
[self.ImagesCacheDictionary setObject:imageDownloaded forKey:key];
[cell.articleImage setImage:[self.ImagesCacheDictionary objectForKey:cell.theKey.description]];
});
}];
[getImageTask resume];
Here is the link to the source code: VTFeeds
Related
Check out AFNetworking an alternative to NSURLSessions